A common way to register in a Laravel API is to send a POST request to /users
containing a username, password, any other info, and also an HMAC signature using a server-side secret. In this example, it’s validated on the server by this class:
public const HASH_ALGORITHM = 'sha256';
protected const REQUEST_KEYS = [
'email',
'name',
];
private $secret;
public function __construct(string $secret = null)
{
if (! $secret) {
throw new \InvalidArgumentException('The registration secret must be provided');
}
$this->secret = $secret;
}
public function verify(Request $request): bool
{
return rescue(
function () use ($request) {
$hash = hash_hmac(
self::HASH_ALGORITHM,
json_encode($request->only(self::REQUEST_KEYS)),
$this->secret
);
return hash_equals(
base64_encode($hash),
$request->get('signature', '')
);
},
false
);
Code language: PHP (php)
So we can see that it’s expecting a Base64-encoded HMAC-SHA256 signature of a JSON array containing the email
and name
properties.
If you’re trying to make this request in Postman, you obviously need to calculate this same signature or it won’t work. Fortunately Postman has pre-request scripts that can inspect bits of your request and environment and generate new elements before your request is sent, and that’s what we need to use.
We don’t want the secret to be saved in our request collection, so we keep it in an environment, and pull it out dynamically when the request is made. Postman includes the Crypto-js package, which includes the necessary signature and encoding functions we need. Coming from PHP, the syntax for these operations feels very convoluted, but it goes like this:
const signature_string = '{"email":"' + request.data.email + '","name":"' + request.data.name + '"}';
const hmac = CryptoJS.HmacSHA256(signature_string, pm.environment.get('REGISTRATION_SECRET'));
const b64 = CryptoJS.enc.Base64.stringify(CryptoJS.enc.Utf8.parse(hmac));
pm.environment.set("REGISTRATION_SIGNATURE", b64);
Code language: JavaScript (javascript)
This builds the string to sign from the request elements we need, calculates the HMAC of it using our secret, and then base64-encodes it before saving it in the Postman environment.
You can then add the signature into your request by adding the REGISTRATION_SIGNATURE
variable to the body:
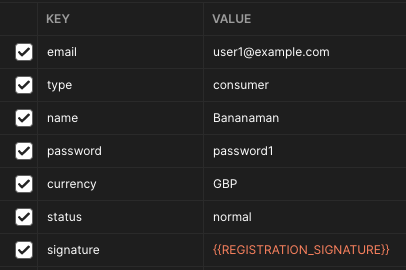
Hope that helps someone!